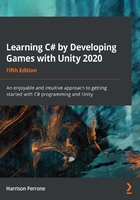
Writing proper C#
Lines of code function like sentences, meaning they need to have some sort of separating or ending character. Every line of C#, called a statement, MUST end with a semicolon to separate them for the code compiler to process.
However, there's a catch that you need to be aware of. Unlike the written word we're all familiar with, a C# statement doesn't technically have to be on a single line; whitespace and newlines are ignored by the code compiler. For example, a simple variable could be written like this:
public int firstName = "Harrison";
Alternatively, it could also be written as follows:
public
int
firstName
=
"Harrison";
These two code snippets are both perfectly acceptable to Visual Studio, but the second option is highly discouraged in the software community as it makes code extremely hard to read. The idea is to write your programs as efficiently and clearly as possible.
There will be times when a statement will be too long to reasonably fit on a single line, but those are few and far between. Just make sure that it's formatted in a way someone else could understand, and don't forget the semicolon.
The second formatting rule you need to drill into your coding muscle memory is the use of curly brackets or braces. Methods, classes, and interfaces all need a set of curly brackets after their declaration. We'll talk about each of these in-depth later on, but it's important to get the standard formatting in your head early on. The traditional practice in C# is to include each bracket on a new line, as shown here:
public void MethodName()
{
}
However, when you create a new script from the Unity Editor or go online to the Unity documentation, you'll see the first curly bracket located on the same line as the declaration:
public void MethodName() {
}
While this isn't something to tear your hair out over, the important thing is to be consistent. "Pure" C# code will always put each bracket on a new line, while C# examples that have to do with Unity and game development will most often follow the second example.
Good, consistent formatting style is paramount when starting in programming, but so is being able to see the fruits of your work. In the next section, we'll talk about how to print out variables and information straight to the Unity console.