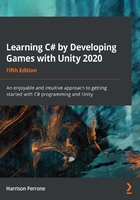
Debugging your code
While we're working through practical examples, we'll need a way to print out information and feedback to the Console window in the Unity editor. The programmatic term for this is debugging, and both C# and Unity provide helper methods to make this process easier for developers. Whenever I ask you to debug or print something out, please use one of the following methods:
- For simple text or inpidual variables, use the standard Debug.Log() method. The text needs to be inside a set of parentheses, and variables can be used directly with no added characters; for example:
Debug.Log("Text goes here.");
Debug.Log(yourVariable);
- For more complex debugging, use Debug.LogFormat(). This will let you place variables inside the printed text by using placeholders. These are marked with a pair of curly brackets, each containing an index. An index is a regular number, starting at 0 and increasing sequentially by 1.
In the following example, the {0} placeholder is replaced with the variable1 value, {1} with variable2, and so on:
Debug.LogFormat("Text goes here, add {0} and {1} as variable
placeholders", variable1, variable2);
You might have noticed that we're using dot notation in our debugging techniques, and you'd be right! Debug is the class we're using, and Log() and LogFormat() are different methods that we can use from that class. More on this at the end of this chapter.
With the power of debugging under our belts, we can safely move on and do a deeper pe into how variables are declared, as well as the different ways that syntax can play out.