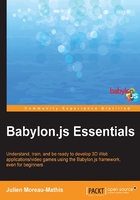
上QQ阅读APP看书,第一时间看更新
Creating your first scene
Now, you have all the necessary elements to build your first scene. Here, the scene will be composed of a rotation camera, point light, and box. Let's create a class using TypeScript and practice with Babylon.js.
Creating a class and the scene nodes
The following class creates the Babylon.js elements directly in the constructor:
export class BasicScene { public camera: BABYLON.ArcRotateCamera; // Our camera public light: BABYLON.PointLight; // Our light public box: BABYLON.Mesh; // Our box private _engine: BABYLON.Engine; // The Babylon.js engine private _scene: BABYLON.Scene; // The scene where to add the nodes // Our constructor. The constructor provides the canvas reference // Then, we can create the Babylon.js engine constructor(canvas: HTMLCanvasElement) { // Create engine this._engine = new BABYLON.Engine(canvas); // Create the scene this._scene = new BABYLON.Scene(this._engine); // Create the camera this.camera = new BABYLON.ArcRotateCamera("camera", 0, 0, 30, BABYLON.Vector3.Zero(), this._scene); this.camera.attachControl(canvas, true); // Create the light this.light = new BABYLON.PointLight("light",new BABYLON.Vector3(20, 20, 20), this._scene); this.light.diffuse = new BABYLON.Color3(0, 1, 0); this.light.specular = new BABYLON.Color3(1, 0, 1); this.light.intensity = 1.0; // Create the box this.box = BABYLON.Mesh.CreateBox("cube", 5, this._scene); } }
Call the runRenderLoop method
Let's add a method to the class that will call the runRenderLoop
method:
public runRenderLoop(): void { this._engine.runRenderLoop(() => { this._scene.render(); }); }
This scene is exactly the same as mentioned in the previous image—there is a box and green light.
Managing the scene graph
To practice with the scene graph, let's create a method that will set the light as a child of the camera. The method will set the light's position at coordinates (x=0
,y=0
,z=0
) and set the parent of the light as the camera:
public setCameraParentOfLight(): void { this.light.parent = this.camera; }