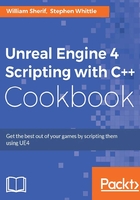
Unmanaged memory – using new/delete
The new
operator is almost the same as a malloc
call, except that it invokes a constructor call on the object created immediately after the memory is allocated. Objects allocated with the operator new
should be deallocated with the operator delete
(and not free()
).
Getting ready
In C++, use of malloc()
was replaced, as best practice, by use of the operator new
. The main difference between the functionality of malloc()
and the operator new
is that new
will call the constructor on object types after memory allocation.

How to do it...
In the following code, we declare a simple Object
class, then construct an instance of it using the operator new
:
class Object { Object() { puts( "Object constructed" ); } ~Object() { puts( "Object destructed" ); } }; Object* object= new Object(); // Invokes ctor delete object; // Invokes dtor object = 0; // resets object to a null pointer
How it works…
The operator new
works by allocating space just as malloc()
does. If the type used with the operator new
is an object type, the constructor is invoked automatically with the use of the keyword new
, whereas the constructor is never invoked with the use of malloc()
.
There's more…
You should avoid using naked heap allocations with the keyword new
(or malloc
for that matter). Managed memory is preferred within the engine so that all memory use is tracked and clean. If you allocate a UObject
derivative, you definitely need to use NewObject< >
or ConstructObject< >
(outlined in subsequent recipes).