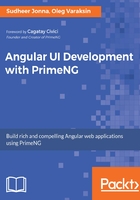
Type definition files
JavaScript programs written in native JavaScript don't have any type information. If you add a JavaScript library such as jQuery or Lodash to your TypeScript-based application and try to use it, the TypeScript compiler can find any type information and warn you with compilation errors. Compile-time safety, type checking, and context-aware code completion get lost. That is where type definition files come into play.
Type definition files provide type information for JavaScript code that is not statically typed. Type definition files ends with .d.ts and only contain definitions which are not emitted by TypeScript. The declare keyword is used to add types to JavaScript code that exists somewhere. Let's take an example. TypeScript is shipped with the lib.d.ts library describing ECMAScript API. This type definition file is used automatically by the TypeScript compiler. The following declaration is defined in this file without implementation details:
declare function parseInt(s: string, radix?: number): number;
Now, when you use the parseInt function in your code, the TypeScript compiler ensures that your code uses the correct types and IDEs show context-sensitive hints when you're writing code. Type definition files can be installed as dependencies under the node_modules/@types directory by typing the following command:
npm install @types/<library name> --save-dev
A concrete example for jQuery library is:
npm install @types/jquery --save-dev
Most of the time, you have the compile target ES5 (generated JavaScript version, which is widely supported), but want to use some ES6 (ECMAScript 2015) features by adding Polyfills. In this case, you must tell the compiler that it should look for extended definitions in the lib.es6.d.ts or lib.es2015.d.ts file. This can be achieved in compiler options by setting the following:
"lib": ["es2015", "dom"]