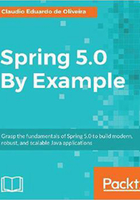
Configuring Swagger
The dependencies are added, now we can configure the infrastructure for Swagger. The configuration is pretty simple. We will create a class with @Configuration to produce the Swagger configuration for the Spring container. Let's do it.
Take a look at the following Swagger configuration:
package springfive.cms.infra.swagger;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.bind.annotation.RestController;
import springfox.documentation.builders.ParameterBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfiguration {
@Bean
public Docket documentation() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.withClassAnnotation(RestController.class))
.paths(PathSelectors.any())
.build();
}
}
The @Configuration instructs the Spring to generate a bean definition for Swagger. The annotation, @EnableSwagger2 adds support for Swagger. @EnableSwagger2 should be accompanied by @Configuration, it is mandatory.
The Docket class is a builder to create an API definition, and it provides sensible defaults and convenience methods for configuration of the Spring Swagger MVC Framework.
The invocation of method .apis(RequestHandlerSelectors.withClassAnnotation(RestController.class)) instructs the framework to handle classes annotated with @RestController.
There are many methods to customize the API documentation, for example, there is a method to add authentication headers.
That is the Swagger configuration, in the next section, we will create a first documented API.