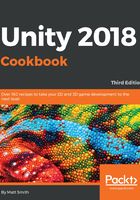
上QQ阅读APP看书,第一时间看更新
How to do it...
To schedule a sound to play after a given delay, do the following:
- Create a new Unity 2D project and import the sound clip files.
- Create a GameObject in the scene containing an AudioSource component linked to the Pacman Opening Song AudioClip. This can be done in a single step by dragging the music clip from the Project panel into either the Hierarchy or Scene panels.
- Repeat the previous step to create another GameObject, containing an AudioSource linked to the Pacman Dies clip.
- For both AudioSources created, uncheck the Play Awake property – so these sounds do not begin playing as soon as the scene is loaded.
- Create a UI Button named Button-music on the screen, changing its text to Play Music Immediately.
- Create a UI Button named Button-dies on the screen, changing its text to Play Dies Sound After 1 second.
- Create an empty GameObject named SoundManager.
- Create a C# script class, DelayedSoundManager, in a new folder, _Scripts, containing the following code, and add an instance as a scripted component to the SoundManager GameObject:
using UnityEngine; public class DelayedSoundManager : MonoBehaviour { public AudioSource audioSourcePacmandMusic; public AudioSource audioSourceDies; public void ACTION_PlayMusicNow() { audioSourcePacmandMusic.Play(); } public void ACTION_PlayDiesSoundAfterDelay() { float delay = 1.0F; audioSourceDies.PlayDelayed(delay); } }
- With the Button-music GameObject selected in the Hierarchy panel, create a new on-click event-handler, dragging the SoundsManager GameObject into the Object slot, and selecting the ACTION_PlayMusicNow() method.
- With the Button-dies GameObject selected in the Hierarchy panel, create a new on-click event-handler, dragging the SoundsManager GameObject into the Object slot, and selecting the ACTION_PlayDiesSoundAfterDelay() method.