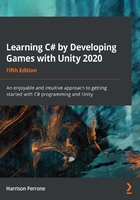
Understanding variable scope
We're getting to the end of our pe into variables, but there's still one more important topic we need to cover: scope. Similar to access modifiers, which determine which outside classes can grab a variable's information, the variable scope is the term used to describe where a given variable exists and its access point within its containing class.
There are three main levels of variable scope in C#:
- Global scope refers to a variable that can be accessed by an entire program; in this case, a game. C# doesn't directly support global variables, but the concept is useful in certain cases, which we'll cover in Chapter 10, Revisiting Types, Methods, and Classes.
- Class or member scope refers to a variable that is accessible anywhere in its containing class.
- Local scope refers to a variable that is only accessible inside the specific block of code it's created in.
Take a look at the following screenshot. You don't need to put this into LearningCurve if you don't want to; it's only for visualization purposes at this point:

When we talk about code blocks, we're referring to the area inside any set of curly brackets. These brackets serve as a kind of visual hierarchy in programming; the farther right-indented they are, the deeper they are nested in the class.
Let's break down the class and local scope variables in the preceding screenshot:
- characterClass is declared at the very top of the class, which means we can reference it by name anywhere inside LearningCurve. You might hear this concept referred to as variable visibility, which is a good way of thinking about it.
- characterHealth is declared inside the Start() method, which means it is only visible inside that block of code. We can still access characterClass from Start() with no issue, but if we attempted to access characterHealth from anywhere but Start(), we would get an error.
- characterName is in the same boat as characterHealth; it can only be accessed from the CreateCharacter() method. This was just to illustrate that there can be multiple, even nested, local scopes in a single class.
If you spend enough time around programmers, you'll hear discussions (or arguments, depending on the time of day) about the best place to declare a variable. The answer is simpler than you might think: variables should be declared with their use in mind. If you have a variable that needs to be accessed throughout a class, make it a class variable. If you only need a variable in a specific section of code, declare it as a local variable.
Note that only class variables can be viewed in the Inspector window, which isn't an option for local or global variables.
With naming and scope in our toolbox, let's transport ourselves back to middle school math class and relearn how arithmetic operations work all over again!