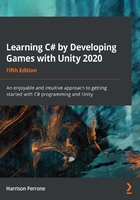
Using access modifiers
Now that the basic syntax is no longer a mystery, let's get into the finer details of variable statements. Since we read code from left to right, it makes sense to begin our variable deep-pe with the keyword that traditionally comes first – an access modifier.
Take a quick look back at the variables we used in the preceding chapter in LearningCurve and you'll see they had an extra keyword at the front of their statements: public. This is the variable's access modifier. Think of it as a security setting, determining who and what can access the variable's information.
Any variable that isn't marked public is defaulted to private and won't show up in the Unity Inspector panel.
If you include a modifier, the updated syntax recipe we put together at the beginning of this chapter will look like this:
accessModifier dataType uniqueName = value;
While explicit access modifiers aren't necessary when declaring a variable, it's a good habit to get into as a new programmer. That extra word goes a long way toward readability and professionalism in your code.
Choosing a security level
There are four main access modifiers available in C#, but the two you'll be working with most often as a beginner are the following:
- Public: This is available to any script without restriction.
- Private: This is only available in the class they're created in (which is called the containing class). Any variable without an access modifier defaults to private.
The two advanced modifiers have the following characteristics:
- Protected: Accessible from their containing class or types derived from it
- Internal: Only available in the current assembly
There are specific use cases for each of these modifiers, but until we get to the advanced chapters, don't worry about protected and internal.
Two combined modifiers also exist, but we won't be using them in this book. You can find more information about them at https://docs.microsoft.com/en-us/dotnet/csharp/language-reference/keywords/access-modifiers.
Let's try out some access modifiers of our own!
Time for action – making a variable private
Just like information in real life, some data needs to be protected or shared with specific people. If there's no need for a variable to be changed in the Inspector window or accessed from other scripts, it's a good candidate for a private access modifier.
Perform the following steps to update LearningCurve:
- Change the access modifier in front of currentAge from public to private and save the file.
- Go back into Unity, select the Main Camera, and take a look at what changed in the LearningCurve section.
Since currentAge is now private, it's no longer visible in the Inspector window and can only be accessed within the LearningCurve script. If we click Play, the script will still work exactly as it did before:

This is a good start on our journey into variables, but we still need to know more about what kinds of data they can store. This is where data types come in, which we'll look at in the next section.