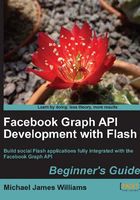
Time for action - retrieving a Page's information in AS3
Set up the project from the Chapter 2 start files, as explained in Chapter 1. Check that the project compiles with no errors (there may be a few warnings, depending on your IDE). You should see a 640 x 480 px SWF, all white, with just three buttons in the top-left corner: Zoom In, Zoom Out, and Reset View:

This project is the basis for a Rich Internet Application (RIA) that will be able to explore all of the information on Facebook using the Graph API. All the code for the UI is in place, just waiting for some Graph data to render. Our job is to write code to retrieve the data and pass it on to the renderers.
I'm not going to break down the entire project and explain what every class does, as the focus of this book is on using Facebook with Flash, not on building RIAs. What you need to know at the moment is a single instance of the controllers.CustomGraphContainerController
class is created when the project is initialized, and it is responsible for directing the flow of data to and from Facebook. It inherits some useful methods for this purpose from the controllers.GCController
class; we'll make use of these later on.
Open the CustomGraphContainerController
class in your IDE. It can be found in \src\controllers\CustomGraphContainerController.as
, and should look like the listing below:
package controllers { import ui.GraphControlContainer; public class CustomGraphContainerController extends GCController { public function CustomGraphContainerController (a_graphControlContainer:GraphControlContainer) { super(a_graphControlContainer); } } }
The first thing we'll do is grab the Graph API's representation of Packt Publishing's Page via a Graph URL, like we did using the web browser. For this we can use a URLLoader
.
Note
The URLLoader
and URLRequest
classes are used together to download data from a URL. The data can be text, binary data, or URL-encoded variables.
The download is triggered by passing a URLRequest
object, whose url
property contains the requested URL, to the load()
method of a URLLoader
.
Once the required data has finished downloading, the URLLoader
dispatches a COMPLETE
event. The data can then be retrieved from its data
property.
Modify CustomGraphContainerController.as
like so (the highlighted lines are new):
package controllers { import flash.events.Event; import flash.net.URLLoader; import flash.net.URLRequest; import ui.GraphControlContainer; public class CustomGraphContainerController extends GCController { public function CustomGraphContainerController (a_graphControlContainer:GraphControlContainer) { super(a_graphControlContainer); var loader:URLLoader = new URLLoader(); var request:URLRequest = new URLRequest(); //Specify which Graph URL to load request.url = "https://graph.facebook.com/PacktPub"; loader.addEventListener(Event.COMPLETE, onGraphDataLoadComplete); //Start the actual loading process loader.load(request); } private function onGraphDataLoadComplete(a_event:Event):void { var loader:URLLoader = a_event.target as URLLoader; //obtain whatever data was loaded, and trace it var graphData:String = loader.data; trace(graphData); } } }
All we're doing here is downloading whatever information is at https://graph.facebook.com/PackPub and tracing it to the output window.
Test your project, and take a look at your output window. You should see the following data:
{"id":"204603129458","name":"Packt Publishing","picture":"http:\/\/profile.ak.fbcdn.net\/hprofile-ak-snc4\/hs302.ash1\/23274_204603129458_7460_s.jpg","link":"http:\/\/www.facebook.com\/PacktPub","category":"Products_other","username":"PacktPub","company_overview":"Packt is a modern, IT focused book publisher, specializing in producing cutting-edge books for communities of developers, administrators, and newbies alike.\n\nPackt published its first book, Mastering phpMyAdmin for MySQL Management in April 2004.","fan_count":412}
If you get an error, check that your code matches the previously mentioned code. If you see nothing in your output window, make sure that you are connected to the Internet. If you still don't see anything, it's possible that your security settings prevent you from accessing the Internet via Flash, so check those.
What just happened?
The line breaks and tabulation between values have been lost, and some characters have been escaped, making it hard to read… but you can see that this is the same data as we obtained when browsing to https://graph.facebook.com/PacktPub. No surprise here; that's all the URLLoader
does.
The data's not very useful to us in that form. In order to do something with it, we need to convert it to an object that we can interact with natively in AS3.
The format which Graph API uses is called JSON (JavaScript Object Notation; pronounced "Jason").
JSON is a human-readable, text-based data format standard. It allows you to represent objects as key-value pairs, like so:
{ "key1": "value1", "key2": "value2", "key3": "value3" }
The values can be strings (enclosed in quote marks), or numbers, Boolean values, or null
(not enclosed in quote marks).
JSON objects can also contain arrays, using square brackets:
{ "key1": "value1", "array": [ "First item in array", "Second item in array", "Third item in array" ] }
They can even contain other JSON objects, by nesting curly braces:
{ "key1": "value1", "subObject": { "subKey1": "subValue1", "subKey2": "subValue2", } }
These sub-objects can contain other objects and arrays, and arrays can contain other objects or arrays, too.
Note that this is very similar to the AS3 syntax for declaring an object:
var as3Object:Object = { key1:"value1", key2:"value2", subObject:{ subKey1:"subValue1" }, myArray:[1, 2, 3] }
Note
For more information, check out http://www.json.org.
Unlike with XML, AS3 has no native features for handling JSON objects—but there is an officially supported library that does.